Reactive programming is an innovative programming style that focuses on handling data flows and changes dynamically. It allows developers to create powerful applications that can efficiently respond to various data events, providing a smooth user experience.
5 Reactive Programming Projects
- Real-time chat application
- Reactive currency exchange service
- Reactive weather forecast application
- Reactive to-do list application
- Reactive e-commerce product catalog
For Java developers, especially those familiar with Java Enterprise Edition (EE), Spring Boot, and REST APIs, mastering reactive programming can significantly enhance your skill set and boost the performance of your applications. In this article, we’ll explore five exciting project ideas to help you dive into reactive programming concepts while developing practical skills.
1. Real-Time Chat Application
Build a real-time chat application where users can send and receive messages instantly. Using Spring WebFlux, you can implement reactive features that allow multiple users to send messages without blocking one another. This project will enhance your knowledge of reactive programming, network protocols and web technologies, culminating in a hands-on experience of creating a fully functional chat application.
Project Details
- Technologies Used: Spring Boot, Spring WebFlux, MongoDB (or any other NoSQL database), Socket.IO for real-time communication.
- Features:
- User authentication and registration for secure access.
- Real-time message broadcasting using reactive streams.
- Save chat history in a database for future reference.
- Skills Learned:
- Understanding of reactive streams and their handling in Spring WebFlux.
- Implementation of WebSocket for real-time communication.
- Experience with non-blocking database operations.
Project Benefits
Through this project, you'll experience the thrill of building a live chat environment. Imagine users engaging in conversations that don’t lag or interrupt! You will also confront challenges like handling multiple connections seamlessly, ensuring that messages reach users without delays, and managing data storage efficiently. You’ll see how reactive programming allows for smooth user interactions, where messages appear instantly, and users can communicate without delays.
2. Reactive Currency Exchange Service
Create a service that provides real-time currency conversion rates using external APIs. The service should reactively update rates without requiring manual refreshes. This project will enhance your understanding of how to work with live data and APIs.
You’ll build a system where users get immediate updates on currency rates. It’s rewarding to see how your application responds to market changes in real-time, offering users the latest information without them having to refresh. You’ll also learn how to optimize retrieval of data for better performance, making your application feel seamless.
Project Details
- Technologies Used: Spring Boot, Spring WebFlux, RestTemplate or WebClient for external API calls, and a simple front-end (optional).
- Features:
- Fetch real-time currency conversion rates.
- Provide endpoints for conversion requests that reactively stream results.
- Implement caching for performance optimization.
- Skills Learned:
- Mastery of asynchronous programming with reactive APIs.
- Handling reactive extensions to manipulate data streams.
- Experience with API integrations and caching strategies.
Getting Started
To begin for this project, follow these steps:
- Set Up Your Development Environment: Ensure you have Java JDK 17 or higher, and Spring Boot dependencies set up in your IDE or project management tool, like Maven or Gradle.
- Understand Reactive Programming Concepts: Familiarize yourself with key concepts of reactive programming, focusing on reactive streams and non-blocking I/O. Spring WebFlux documentation is a great resource for understanding these concepts.
- Choose an External API: Select a reliable currency exchange API, such as ExchangeRate-API, Open Exchange Rates, or any other that allows free access for development and read through their documentation for API access and usage.
Project Steps
1. Setting Up Your Project
Go to Spring and create a new Spring Starter Project:
Next, fill in your project details (name, package, etc.) and select dependencies:
- Include Spring WebFlux
- Optionally, include Spring Boot DevTools for hot reloading.
2. Generate the Project
Click on the GENERATE
button and download the sample code as zip. Unzip it and open it in your favorite IDE, such as IntelliJ IDEA, Eclipse or VS Code.
3. Set Up Your Application Properties
Configure your application.properties file to include any necessary settings for your external currency API, such as the API base URL and access keys.
4. Create API Interface for External Currency Service
Implement an interface using the WebClient or RestTemplate to fetch real-time currency conversion rates from the external API.
5. Build the Reactive Service
Create a service class that uses the API interface to reactively retrieve currency rates and provide endpoints for currency conversion requests.
6. Implement Caching Mechanism
Utilize appropriate caching strategies, such as using ConcurrentHashMap
or a caching library, to store fetched currency rates and reduce the number of API calls.
7. Create Endpoints
Set up controller classes to expose endpoints for fetching conversion rates and handling conversion requests
8. Testing the Application
Write unit tests and integration tests to validate the functionality of your service and ensure that it handles API rate limits, caching and error scenarios effectively.
9. Running Your Application
Run your Spring Boot application either through your IDE or by using the command line. Access the REST endpoints to observe how your service works in real-time.
10. Project Structure
The currency exchange project follows a standard Spring Boot project layout, organized with Maven. Below is the structure as seen in my IDE, which provides a clear separation of concerns for configuration, services, controllers and response models
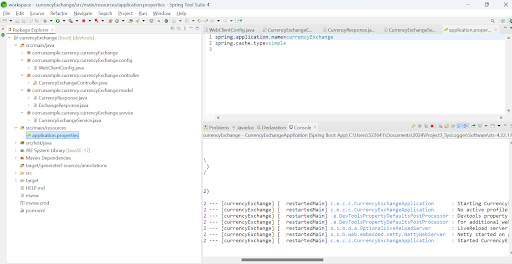
src/main/java/com/example/currency/currencyExchange/
: Contains the core application code.config/WebClientConfig.java
: Configures the WebClient with an insecure SSL context for reactive HTTP requests.controller/CurrencyExchangeController.java
: Defines the REST endpoint (/api/v1/currency/convert
) to handle currency conversion requests.service/CurrencyExchangeService.java
: Implements the business logic, fetching exchange rates reactively and caching results.response/
: Holds data models like CurrencyResponse (for the external API response) and ExchangeResponse (for the API output).pom.xml
: Maven configuration with dependencies like Spring Boot WebFlux and Reactor Netty..gitignore
: Excludes build artifacts, liketarget/
, IDE files, like.idea/
, and logs.README.md
: Provides setup instructions and project details.
11. Test with Postman
Open Postman and create a new request by clicking the “New”
button or selecting an existing workspace.
Then, enter the full URL with query parameters. Assuming your app runs on https://localhost:8080
, the URL will look like:
https://localhost:8080/api/v1/currency/convert?from=USD&to=EUR&amount=100
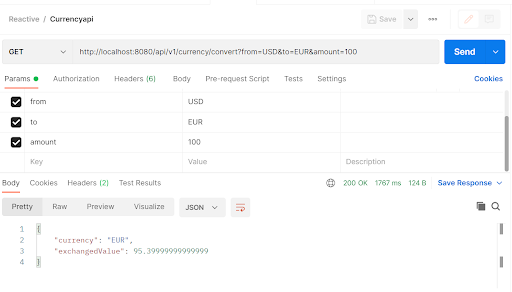
Why This Project Good for Practicing Reactive Programming
- Asynchronous Data Handling: This project harnesses the power of asynchronous programming. You will gain hands-on experience managing and processing data flows concurrently.
- Real-Time Data Updates: You will learn to implement real-time features that respond dynamically to user input, which is a core aspect of reactive programming.
- Integration with External Services: You’ll practice integrating third-party APIs, learning how to handle responses and errors reactively.
Common Challenges
- API Rate Limiting: Most external APIs enforce rate limits. You’ll need to handle scenarios where your application may exceed the limit. Implement backoff strategies to manage API calls effectively.
- Data Caching: Designing an effective caching mechanism to avoid excessive calls to the currency API can be tricky. You’ll need to ensure that you provide timely updates while managing cache size and expiration.
- Reactive Programming Concepts: If you’re new to reactive programming, you may initially find the concepts of observables, subscribers, and schedulers challenging. Familiarizing yourself with these concepts will be crucial as you implement your project.
3. Reactive Weather Forecast Application
Picture building an application where users can get immediate weather updates. As you work on this project, you will appreciate the importance of speedy data retrieval. You’ll enjoy the challenge of visualizing the forecast, presenting it dynamically, and seeing firsthand how users interact with live data. You’ll appreciate how reactive programming allows users to see immediate updates, enhancing their overall experience.
Project Details
- Technologies Used: Spring Boot, Spring WebFlux, a weather API (like OpenWeatherMap), Thymeleaf or any front-end framework.
- Features:
- An endpoint to get weather details based on user input.
- Real-time updates on weather conditions using reactive streams.
- Optional: Visualization of data through charts or graphs.
- Skills Learned:
- Building non-blocking applications with Spring WebFlux.
- Integrating third-party APIs with reactive programming techniques.
- Enhancing UI responsiveness with reactive data handling.
Project Benefits
Creating a weather forecasting service allows you to practice integrating APIs with a focus on asynchronous data retrieval, showcasing reactive programming’s strengths in enhancing user experience.
4. Reactive To-Do List Application
Build a to-do list application that allows users to manage tasks through a reactive interface where updates reflect immediately. Imagine users adding or completing tasks, with changes appearing instantly for everyone. You’ll tackle real-world challenges like managing concurrent updates and ensuring a smooth user experience, making it feel like a living application.
Project Details
- Technologies Used: Spring Boot, Spring WebFlux, MongoDB, React (or Angular) for the front-end.
- Features:
- Simplified CRUD operations for managing tasks.
- Real-time updates reflected on the UI using WebSockets or Server-Sent Events (SSE).
- User authentication and task categorization.
- Skills Learned:
- Experience with CRUD operations in a reactive stack.
- Handling real-time updates and changes smoothly in the user interface.
- Improved understanding of building responsive and interactive UIs.
Project Benefits
This project exemplifies the use of reactive programming in building practical applications with real-time features, reinforcing concepts learned throughout your development journey.
5. Reactive E-commerce Product Catalog
Create a product catalog for an e-commerce application, allowing users to view, search and filter products reactively. This project allows you to create a vibrant shopping experience. Users can search for products and see results instantly, bringing your catalog to life. You’ll enjoy the challenge of implementing powerful filtering and sorting capabilities while navigating the complexities of handling user data and feedback in real-time.
Project Details
- Technologies Used: Spring Boot, Spring WebFlux, PostgreSQL, Elasticsearch (optional for advanced searching), and a front-end framework.
- Features:
- Endpoint for fetching product details.
- Real-time search and filtering capabilities.
- User reviews and ratings displayed reactively.
- Skills Learned:
- Implementing reactive data handling for large datasets.
- Experience with search algorithms and filtering in a reactive manner.
- Gaining knowledge about integrating multiple tools and technologies for a functional application.
Project Benefits
This project unifies various concepts in reactive programming, showcasing the power of responsive applications in the field of e-commerce.
Advantages to Experimenting With Reactive Programming Projects
Engaging in these five reactive programming projects will not only enhance your skills but also provide you with valuable insights into building modern web applications. Whether you're looking to deepen your knowledge of Spring Boot, improve your API integration skills, or simply enjoy coding as a hobby, these projects will set you on the path to mastering reactive programming in Java.